Apache tucsany provides a framework for developing SOA solution that is based on Service Component Architecture (SCA) standard. Tucsany offers following advantages:
1) Provides a model for creating composite applications by defining the services in the fabric and their relationships with one another.
2) Enables service developers to create reusable services that only contain business logic.
3) Existing applications can work with new SCA compositions. This allows for incremental growth towards a more flexible architecture, outsourcing or providing services to others.
Tucsany implemented in java and c++ programming language. For more information visit apache tucsany site.
In the growing uses of business rules in SOA, on the current post i decide to make a laboratory work to show how to use business rules in SCA SOA solution.
For more information to get benifit from business rules please consider my some previous post about Ilog business rules.
On the current tutorial we are going to use following tools and libraries:
1) Maven 2.0.9
2) JAX-WS 2.1.5
3) Tucsany SCA 1.4
4) Ilog Jrules 6.0.7
All the information to download and install of all tools and frameworks should be found on their home site.
For developing the tutorial i preferred to use Intelli Idea 7. But you can use your own IDE because we uses maven to manage the project and you can easily create any particullar project by maven tools.
Create composite service application:
The following shows the composition diagram for the composite service application we are about to create.
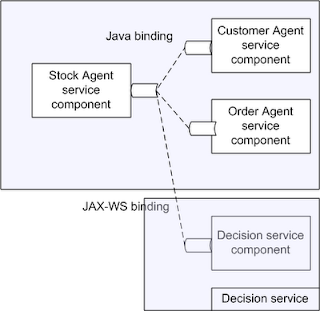
The composite stock agent component consist of two services and one decision service. There is Customer agent service, from where you might get all the necessary information of customer by customer id. From the otder service we will get product information and sned all the information to decision service for some validation. If outgoing status approved, then stock agent generate products bill. Actually example used here to only show the jax-ws binding to ilog decision service.
Now we start developing from the scratch by using maven tools. First we will start ilog jrules server and deploy the example decision service provide with the jrules installation. If you deploy it's properly, you should get the wsdl in the folowing location
http://localhost:8080/DecisionService/ws/PreTradeChecksRuleApp/1.0/PreTradeChecks/1.0?WSDL
For more information develop and deploy decesion service on Jrules, please refer to the following post.
Now it's time to create the pom.xml file as follows:
First of all we build the project name sca-simple by maven tools and append all the necessary dependency on it. Download the wsdl file to the src\main\resources folder to generate proxy releated class from the decesion service. In the current project i use latest version of JAX-WS which you probably need to download and install manually on the local maven repositories.
Here is the command to install libraries manually on the maven local repositories.
Project is now ready to coding. If we will run the following command in console,
$mvn clean compile
the maven will first generate all the stub class from the wsdl and compile the project. This may take a few minutes because maven will download a lot of tuscany modules and files to your local repositories.
For simplicity we will only take a look to the main parts of the project. Anyway the source code the project will be downlodable.
First we will create interface for the decision service as follows:
and also the implemention:
Finally we will create composite xml file to compose all the component.
At these moment all the component is ready to compose. You can build and run the program by maven as follows:
$mvn clean compile
$mvn exec:java
1) Provides a model for creating composite applications by defining the services in the fabric and their relationships with one another.
2) Enables service developers to create reusable services that only contain business logic.
3) Existing applications can work with new SCA compositions. This allows for incremental growth towards a more flexible architecture, outsourcing or providing services to others.
Tucsany implemented in java and c++ programming language. For more information visit apache tucsany site.
In the growing uses of business rules in SOA, on the current post i decide to make a laboratory work to show how to use business rules in SCA SOA solution.
For more information to get benifit from business rules please consider my some previous post about Ilog business rules.
On the current tutorial we are going to use following tools and libraries:
1) Maven 2.0.9
2) JAX-WS 2.1.5
3) Tucsany SCA 1.4
4) Ilog Jrules 6.0.7
All the information to download and install of all tools and frameworks should be found on their home site.
For developing the tutorial i preferred to use Intelli Idea 7. But you can use your own IDE because we uses maven to manage the project and you can easily create any particullar project by maven tools.
Create composite service application:
The following shows the composition diagram for the composite service application we are about to create.
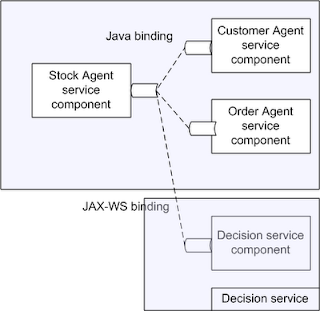
The composite stock agent component consist of two services and one decision service. There is Customer agent service, from where you might get all the necessary information of customer by customer id. From the otder service we will get product information and sned all the information to decision service for some validation. If outgoing status approved, then stock agent generate products bill. Actually example used here to only show the jax-ws binding to ilog decision service.
Now we start developing from the scratch by using maven tools. First we will start ilog jrules server and deploy the example decision service provide with the jrules installation. If you deploy it's properly, you should get the wsdl in the folowing location
http://localhost:8080/DecisionService/ws/PreTradeChecksRuleApp/1.0/PreTradeChecks/1.0?WSDL
For more information develop and deploy decesion service on Jrules, please refer to the following post.
Now it's time to create the pom.xml file as follows:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.blu.sca.simple</groupId> <artifactId>sca-simple</artifactId> <packaging>jar</packaging> <version>1.0-SNAPSHOT</version> <name>sca-simple</name> <url>http://maven.apache.org</url> <repositories> <repository> <id>apache.incubator</id> <url>http://people.apache.org/repo/m2-incubating-repository</url> </repository> </repositories> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <dependency> <groupId>org.apache.tuscany.sca</groupId> <artifactId>tuscany-node-api</artifactId> <version>1.4</version> </dependency> <dependency> <groupId>org.apache.tuscany.sca</groupId> <artifactId>tuscany-node-impl</artifactId> <version>1.4</version> </dependency> <dependency> <groupId>org.apache.tuscany.sca</groupId> <artifactId>tuscany-implementation-java-runtime</artifactId> <version>1.4</version> </dependency> <dependency> <groupId>org.apache.tuscany.sca</groupId> <artifactId>tuscany-domain-manager</artifactId> <version>1.4</version> </dependency> <dependency> <groupId>org.apache.tuscany.sca</groupId> <artifactId>tuscany-binding-sca</artifactId> <version>1.4</version> </dependency> <dependency> <groupId>org.apache.tuscany.sca</groupId> <artifactId>tuscany-binding-sca-axis2</artifactId> <version>1.4</version> </dependency> <dependency> <groupId>org.apache.tuscany.sca</groupId> <artifactId>tuscany-host-embedded</artifactId> <version>1.4</version> </dependency> <dependency> <groupId>javax.xml.ws</groupId> <artifactId>jaxws-api</artifactId> <version>2.1.5</version> </dependency> <dependency> <groupId>javax.xml.bind</groupId> <artifactId>jaxb-api</artifactId> <version>2.1.5</version> </dependency> <dependency> <groupId>com.sun.xml.ws</groupId> <artifactId>jaxws-rt</artifactId> <version>2.1.5</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>jaxws-maven-plugin</artifactId> <executions> <execution> <!--<phase>process-resources</phase>--> <goals> <goal>wsimport</goal> </goals> </execution> </executions> <configuration> <packageName>com.blu.sca.dservice</packageName> <keep>true</keep> <sourceDestDir>${basedir}/src/main/java</sourceDestDir> <wsdlDirectory>${basedir}/src/main/resources</wsdlDirectory> <wsdlFiles> <wsdlFile>pretrade.wsdl</wsdlFile> </wsdlFiles> </configuration> <dependencies> <groupId>com.sun.xml.ws</groupId> <artifactId>jaxws-tools</artifactId> <version>2.1.5</version> </dependencies> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.5</source> <target>1.5</target> <encoding>UTF-8</encoding> </configuration> </plugin> <plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>exec-maven-plugin</artifactId> <version>1.1</version> <executions> <execution> <goals> <goal>java</goal> </goals> </execution> </executions> <configuration> <mainClass>com.blu.sca.simple.agent.decision</mainClass> </configuration> </plugin> </plugins> <resources> <resource> <directory>src/main/resources</directory> <includes> <include>**/*</include> </includes> <filtering>true</filtering> </resource> </resources> </build> </project>
First of all we build the project name sca-simple by maven tools and append all the necessary dependency on it. Download the wsdl file to the src\main\resources folder to generate proxy releated class from the decesion service. In the current project i use latest version of JAX-WS which you probably need to download and install manually on the local maven repositories.
Here is the command to install libraries manually on the maven local repositories.
mvn install:install-file -Dfile=D:\Distributors\java\webservice\jax-ws\jaxws-ri\lib\jaxws-rt.jar -DgroupId=com.sun.xml.ws -DartifactId=jaxws-rt -Dversion=2.1.5 -Dpackaging=jar -DgeneratePom=true
Project is now ready to coding. If we will run the following command in console,
$mvn clean compile
the maven will first generate all the stub class from the wsdl and compile the project. This may take a few minutes because maven will download a lot of tuscany modules and files to your local repositories.
For simplicity we will only take a look to the main parts of the project. Anyway the source code the project will be downlodable.
First we will create interface for the decision service as follows:
package com.blu.sca.simple.services; import org.osoa.sca.annotations.Remotable; @Remotable public interface IRulesService { String lookup(Customer customer, Product product); }
and also the implemention:
package com.blu.sca.simple.impl; import com.blu.sca.simple.services.IRulesService; import com.blu.sca.rules.*; import com.sun.org.apache.xerces.internal.jaxp.datatype.XMLGregorianCalendarImpl; import org.osoa.sca.annotations.Reference; import org.osoa.sca.annotations.AllowsPassByReference; import org.osoa.sca.annotations.Service; import org.osoa.sca.ServiceRuntimeException; import javax.xml.datatype.XMLGregorianCalendar; import javax.xml.bind.JAXBContext; import javax.xml.bind.Marshaller; import java.io.StringWriter; @Service(IRulesService.class) @AllowsPassByReference public class RulesService implements IRulesService { @Reference protected DecisionServicePreTradeChecks dsrService; public String lookup(Customer customer, Product product) { // create request DecisionServiceRequest request = new DecisionServiceRequest(); CustomerParameter customarP = new CustomerParameter(); customarP.setCustomer(customer); OrderParameter orderP = new OrderParameter(); orderP.setOrder(product); request.setCustomerParameter(customarP); request.setOrderParameter(orderP); DecisionServiceResponse response = null; String str=""; ReportParameter report=null; try{ response = dsrService.executeDecisionService(request); report = response.getReportParameter(); str = report.getOrder().getStatus(); }catch(DecisionServiceSoapFault fault){ str = fault.getMessage()); } return str; } }
Finally we will create composite xml file to compose all the component.
<?xml version="1.0" encoding="UTF-8"?> <composite xmlns="http://www.osoa.org/xmlns/sca/1.0" targetNamespace="http://scadecision" xmlns:sample="http://scadecision" name="Decisionservice"> <component name="BookingAgentServiceComponent"> <implementation.java class="com.blu.sca.simple.impl.BookingAgentServiceComponent"/> <reference name="customerService" target="CustomerServiceComponent" /> <reference name="orderService" target="OrderServiceComponent" /> <reference name="rulesService" target="RulesServiceComponent" /> </component> <component name="RulesServiceComponent"> <implementation.java class="com.blu.sca.simple.impl.RulesService"/> <reference name="dsrService"> <binding.ws uri="http://localhost:8080/DecisionService/ws/PreTradeChecksRuleApp/1.0/PreTradeChecks/1.0?WSDL" /> </reference> </component> <component name="CustomerServiceComponent"> <implementation.java class="com.blu.sca.simple.impl.CustomerService"/> </component> <component name="OrderServiceComponent"> <implementation.java class="com.blu.sca.simple.impl.OrderService"/> </component> </composite>
At these moment all the component is ready to compose. You can build and run the program by maven as follows:
$mvn clean compile
$mvn exec:java
Comments